jQuery uploading widget
- We’ve built the next version of the File Uploader.
Uploadcare jQuery widget is responsive and mobile-ready HTML5 file uploader that allows users to select and upload multiple files from various sources. Also, it includes an in-browser image editor. You can customize the appearance and functionality to match your website and task.
Uploading Widget is supplied as a JavaScript library. It overrides an <input type="file">
control on an HTML page with a button that opens up the Uploading Widget dialog.
Like this:
Uploading Widget Features
Uploading Widget helps you perform the following tasks:
- Uploading
- Add a file uploading capability to your website or app.
- Upload files of any type and up to 5 TB in size.
- Get files from various upload sources, including local storage, camera, social media, and cloud storage services.
- Upload multiple files in one go.
- Track upload jobs with an individual progress bar for each file.
- Speed up the uploading with the uploading network (it works like CDN).
- Image Handling
- Show image previews.
- Implement custom image crop options.
- Edit, enhance, and apply photo filters to images in any browser.
- Validation
- Validate files by their format or size.
- Validate files by their MIME type (server-side filtering).
- Automatically resize large incoming images.
- Security
- Make your uploading system compatible with SOC 2, HIPAA, and more.
- Prevent remote code execution through File Uploading.
- Prevent code execution in uploaded files like
SVG
,html
andxml
.
- Reliability
- All your uploads go to the storage covered by SLA with a 99.9% uptime.
Supported browsers
The current Uploading Widget (v3) works in all modern browsers, desktop and mobile. Here's a list of supported browsers:
Desktop | Mobile |
---|---|
Chrome: 37+ | Android Browser: 4.4+ |
Firefox: 32+ | Opera Mobile: 8+ |
Safari: 9+ | iOS Safari: 9+ |
Edge: 12+ | IE Mobile: 11+ |
IE: 10+ | Opera Mini: Last |
Uploading Widget will most probably run in older browser versions as well. In case you need legacy browser support (IE8), try out Uploading Widget v2.
More on browser version support.
Installation
Select either option to install Uploading Widgetr:
Refer to no-code integrations to use Uploading Widget with your website platform like Shopify, etc.
Before proceeding with your install, check out the dependencies and Uploading Widget bundles below.
Dependencies
Uploadcare Uploading Widget doesn't have any external dependencies except for jQuery. Generally, Uploading Widget comes in two versions: with and without jQuery.
For example, you can use jQuery commands on the page if you included a bundle with jQuery:
var $ = uploadcare.jQuery;
$('body').append('It works!');
Bundles
Depending on your project, you can select a specific Uploading Widget JS library bundle:
uploadcare.full.js
— a full bundle with built-in jQueryuploadcare.js
— a default bundle without jQueryuploadcare.api.js
— a bundle without Uploading Widget UI and jQuery JavaScript API onlyuploadcare.ie8.js
— a full bundle with built-in jQuery 1.x for IE 8 support (widget v.2.x and earlier)uploadcare.lang.en.js
— a bundle without jQuery,en
locale only
Include a minified bundle version by adding .min
before .js
.
By default, minified (and without jQuery) uploadcare.min.js
is exported to NPM
and other package managers.
Global installation
Get your public API key and
include this into the <head>
:
<script>
UPLOADCARE_PUBLIC_KEY = 'YOUR_PUBLIC_KEY';
</script>
<script src="https://ucarecdn.com/libs/widget/3.x/uploadcare.full.min.js"></script>
Note: If you already use jQuery, you can use the alternative bundle that comes without jQuery, so you won't download it twice.
Now you can use the Uploader:
<input type="hidden" role="uploadcare-uploader" name="my_file_input" />
NPM
npm install uploadcare-widget
import uploadcare from 'uploadcare-widget'
You can get a Uploading Widget instance and configure it with configuration object:
<input id="uploader" type="hidden" />
const widget = uploadcare.Widget("#uploader", { publicKey: 'YOUR_PUBLIC_KEY' });
Configure
Set of features, such as upload sources, image editing tools, can be customized via Uploading Widget options. You can have mixed settings for different Uploading Widget instances. Global variables will affect all File Uploader instances, and local attributes will override global settings.
Here's how you can configure Uploading Widget:
- Dashboard with a web UI. It generates code that you can use on your page or share a link to a colleague with your config.
- Global variables, initialized on page load.
- Local attributes, initialized when a new Uploading Widget instance is created.
- The
settings
object.
Global variables
Globals are specified as global JavaScript variables in your <script>
tag.
For example:
<script>
UPLOADCARE_PUBLIC_KEY = 'YOUR_PUBLIC_KEY';
UPLOADCARE_LOCALE = 'ru';
UPLOADCARE_CLEARABLE = true;
</script>
Local attributes
Local options are specified in the target <input>
tag as data-*
attributes.
For example:
<input type="hidden" role="uploadcare-uploader"
data-public-key="YOUR_PUBLIC_KEY"
data-images-only
/>
When setting boolean options locally in HTML tag attributes, any value or no
value is considered as true
:
<input data-option="true" />
<input data-option="any-value" />
<input data-option="" />
<input data-option />
To disable a local option, use either:
<input data-option="false" />
<input data-option="disabled" />
Settings object
Most of the Uploading Widget options can also be set within the settings
object.
See the Uploading jQuery Widget API reference for more details.
For example:
<input id="uploader" type="hidden" />
const widget = uploadcare.Widget("#uploader", {
publicKey: 'YOUR_PUBLIC_KEY',
imagesOnly: true,
crop: '300x200'
});
Upload sources
Uploading Widget supports more than a dozen of upload sources, including local file storage, web camera; external URL; cloud services, and social networks. In UI, the sources are shown as tabs.
The set of enabled upload sources is controlled via the data-tabs
option.
List of supported Upload Sources
Code | File Source | Default |
---|---|---|
file | Local disk | On |
camera | Local webcam | On |
url | Any URL | On |
facebook | On | |
gdrive | Google Drive | On |
gphotos | Google Photos | On |
dropbox | Dropbox | On |
instagram | On | |
evernote | Evernote | On |
flickr | Flickr | On |
onedrive | OneDrive | On |
box | Box | Off |
vk | VK | Off |
huddle | Huddle | Off |
Configuring Upload Sources
You can configure the set of upload sources globally or per Uploading Widget
instance. The global parameter is called UPLOADCARE_TABS
. Locally you can
utilize the data-tabs
attribute.
In both cases, you'll pass a space-separated string with tab names.
Configuring the set of sources globally:
<script>
UPLOADCARE_TABS = 'url file facebook';
</script>
Configuring the list of sources locally:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-tabs="url file facebook"
/>
Custom tabs
You can add custom tabs into your Uploading Widget. These tabs can be additional upload sources or whatever you design them to be. For example, display all uploaded files.
Registering a new tab
Register a new tab via the registerTab
method.
<div id="uploader-placeholder">Uploading. Please wait...</div>
<script>
function favoriteFiles(container, button, dialogApi, settings) {
...
}
uploadcare.registerTab('favorites', favoriteFiles);
uploadcare.openPanel('#uploader-placeholder', null, {
tabs: 'favorites file facebook dropbox gdrive instagram vk',
favoriteFiles: [...]
});
</script>
Coding tab's actions
Once the tab is registered, write a custom code. The following code will display
uploaded images made with this Uploading Widget instance. It'll pass a list of file
UUIDs with the settings
object. When a user
selects a file for uploading, the file info can be passed to the dialog using
dialogApi
.
function favoriteFiles(container, button, dialogApi, settings) {
$.each(settings.favoriteFiles, function(i, uuid) {
container.append($('<img>', {
'class': 'favorite-files-image',
'src': settings.cdnBase + '/' + uuid + '/-/scale_crop/280x280/center/',
})
.on('click', function(e) {
dialogApi.addFiles([uploadcare.fileFrom('uploaded', uuid, settings)])
})
);
});
}
Adjusting the look
Customize your custom tab's look via CSS. Use <svg>
and <symbol>
elements:
<svg width="0" height="0" style="position:absolute">
<symbol id="uploadcare--icon-favorites" viewBox="0 0 32 32">
<path d="M 16 22.928 L 23.416 27.4 L 21.454 18.965 L 28 13.292 L 19.37 12.552 L 16 4.6 L 12.629 12.552 L 4 13.292 L 10.546 18.965 L 8.584 27.4 Z"/>
</symbol>
</svg>
.uploadcare--menu__item_tab_favorites.uploadcare--menu__item_current {
color: #f0cb3c;
}
Custom tab in action
Here’s a live example of the Uploading Widget with the custom tab we've just created. It displays images uploaded with this Uploading Widget instance:
Multiple File Uploading
Uploadcare Uploading Widget allows you to upload multiple files in one go. Each file will have its tiny progress bar and a preview when it's uploaded.
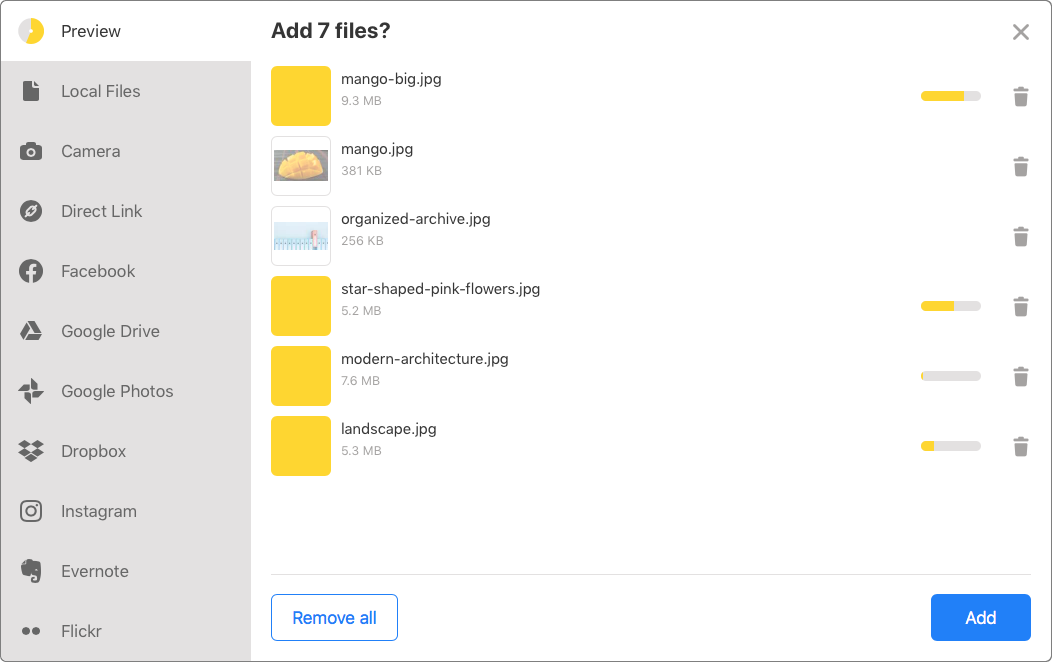
Uploading Widget will display individual errors if some files couldn't be uploaded (e.g., due to size or format validation failure) and it won't affect the rest of the upload.
Enable batch uploading
Enable batch file uploading with the data-multiple
attribute
in the Uploading Widget <input>
element.
<input type="hidden" role="uploadcare-uploader" name="my_files"
data-multiple="true"
/>
Check out multiple file uploading:
Multiple file uploads are collected as file groups with
respective group_id
as opposed to single file UUIDs.
Automatically resize uploaded images
Uploadcare Uploading Widget lets you accept hi-res images and shrink them in size to a reasonable resolution, keeping the original aspect ratio.
Benefits of automatic image resize on upload:
- Users don't need to downscale images on their devices to meet the uploading requirements.
- Optimized storage.
- Faster uploading.
Use the data-image-shrink
option to apply
client-side image resize with values like:
800x600
, shrinks images to 0.48 megapixels with the default JPEG quality of 80% (default, when not set).1600x1600 95%
, shrinks images to 2.5 megapixels with the JPEG quality set to 95%.
Specs and limits
The output resolution limit for data-image-shrink
is 268 MP (e.g., 16384x16384
).
It conforms to the maximum resolution that WebKit desktop browsers support.
We recommend not to use values greater than 16.7 MP (4096x4096
),
because it's a current limit for iOS devices.
Uploaded images won't be shrunk in the following cases:
- When a client browser doesn't support a specified output resolution.
- For images uploaded from social media and URLs.
- If the
original resolution
is less than 2x larger than thetarget resolution
. For example, it won't shrink a 2560x1560px (4 MP) image to 1600x1600px (2.5 MP). It will work if you had a 2448x3264px (8 MP) input image. This limitation preserves an optimal image quality and file size balance. - If the image color mode is CMYK.
The output format will be JPEG by default unless your input image has an alpha channel (transparency). In this case, PNG will be used instead. Grayscale images will be converted to RGB.
EXIF and ICC profile info is copied as-is and includes an original image orientation, camera model, geolocation, and other settings of an original image.
Resize to 1 MP on a client side:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-preview-step=""
data-image-shrink="1024x1024"
/>
Resize multiple files to 0.4 MP on a client side:
<input type="hidden" role="uploadcare-uploader" name="my_files"
data-multiple=""
data-image-shrink="640x480"
/>
Localization
Uploadcare Uploading Widget is highly customizable and implements UI localization and custom pluralization rules. With 31 locales, you can make your app instantly adapt to user languages.
There currently are: ar
az
ca
cs
da
de
el
en
es
et
fr
he
is
it
ja
ko
lv
nb
nl
pl
pt
ro
ru
sk
sr
sv
tr
uk
vi
zhTW
zh
You can either set an existing locale or add a custom one along with its pluralization rules.
Adding a locale
You can add your localization, if there's no one yet, by forking the main Uploading Widget repo and adding a new localization file to this list.
Another option is overriding specific locale items in your global Uploading Widget configuration:
UPLOADCARE_LOCALE = 'en';
UPLOADCARE_LOCALE_TRANSLATIONS = {
buttons: {
cancel: 'Cancel',
remove: 'Remove',
choose: {
files: {
one: 'Choose a file',
other: 'Pick files'
},
images: {
one: 'Choose an image',
other: 'Load images'
}
}
}
};
The default is an English locale. If a string item is missing in a locale you created or customized, English will be a fallback.
Uploading errors can also be redefined in the locale. You can see the reference here.
Pluralization rules
Pluralization rules may vary in different languages. In the English locale,
there'll be "1 file"
, but "3 files"
. This rule is described under the
file:
key in the locale file.
Strings with quantitative values are based on what a pluralization function returns. You'll pass a number into a function, and it'll output a subkey related to your input.
function pluralize(number) {
// do something
return 'some_subkey';
}
There are two subkeys for the English localization: one
and the other
.
However, it can get more complex with other languages. For example, take a look
at the file:
subkeys for the Russian locale.
The %1
sequence is used to format numbers into pluralized strings.
Each locale we provide with the Uploading Widget is supplied with its Unicode-based
pluralization rules. If you wish to override those,
you can define a custom pluralization function and assign it to the
UPLOADCARE_LOCALE_PLURALIZE
variable.
The following setting makes the Uploading Widget use the message under the some
subkey for input numbers from 2 to 10:
UPLOADCARE_LOCALE_TRANSLATIONS = {
file: {
one: 'Only one file! :(',
some: 'Only %1 files. Upload more.',
many: '%1 files!! That\'s a lot.'
}
};
UPLOADCARE_LOCALE_PLURALIZE = function(n) {
if (n === 1) return 'one';
if (n > 1 && n <= 10) return 'some';
return 'many';
};
Styling
Uploadcare jQuery widget can be easily integrated into your product and match your website look or a web app's UI.
Scaling Uploading Widget Elements
The Uploading Widget is designed to inherit styles from your page organically: dialog elements get scaled in line with your font size:
Times New Roman, 12px:
Courier New, 18px:
Styling With CSS
The jQuery widget is thoroughly annotated with CSS classes. It's your starting point into deeper customization. You can find a class for every Uploading Widget item by inspecting its elements or sifting through the File Uploader source code.
The Uploading Widget dialog window look can be customized via the
uploadcare--dialog
class.
Changing Uploader Button Color
Changing the button color is one of the most common cases:
Button Shadow
You can add shadow and experiment with fonts and colors:
Uploading Circle Color
You can display the file uploading progress. The fill color can be changed via
the CSS color
property, while border-color
will work for your background.
Here, you can test the Uploading Widget with a customized uploading circle:
Custom Progress Bar
You can replace the built-in progress bar. To do that, you need to add a
listener to the current Uploading Widget instance and get it in the onChange
callback. It'll be a file object for regular Uploading Widget or a group object
for multiple Uploading Widgets. After that, listen to the progress
event and
change your progress bar according to the current uploadProgress
.
The following installProgressBar
function does all that. It receives the two
arguments: the Uploading Widget instance and a progress bar DOM element. Everything
else runs on CSS, animation included.
Uploaded Image Preview
The default Uploading Widget behavior is to show an image preview when a user selects an image. You might want to embed this preview on your page somewhere around the Uploading Widget button. Such a preview could be more informative than simply displaying file names and sizes.
Note, you have full control over the size and position of your embed. Just use CSS.
Image preview for a multi-file Uploading Widget may look differently:
You can change the displayed images or rearrange the existing ones; all changes will then be reflected in the thumbnail list.
Uploading Widget Embed
User experience means the world to us. Therefore, we provide a lot of customization options that cover both Uploading Widget appearance and behavior.
The look of the Uploading Widget can be changed via CSS, and Dashboard is a great starting point for controlling your Uploading Widget behavior.
Another thing you can do is to embed the Uploading Widget as a panel as opposed to a default dialog window.
Embed Uploading Widget Using Panel
By default, the Uploading Widget dialog appears on a button click. The dialog will appear in a lightbox, which overlays your page's content and dims the background.
However, you might want to show the Uploading Widget interface right away. This
appearance is named panel
.
<div id="uploader-placeholder">Uploading. Please wait...</div>
<script>uploadcare.openPanel('#uploader-placeholder');</script>
The snippet above replaces your DOM element with the uploadcare-placeholder
ID
and puts it in place once a user selects a file. This can be used to indicate
the uploading process. Also, the panel can be closed by simply selecting a file.
Panel Styling
Similar to the Uploading Widget dialog, the panel can be customized.
The appearance of your embed can be changed via CSS. In this example, we remove a sharp border:
#uploader-styling {
margin-top: 10px;
}
#uploader-styling .uploadcare--panel,
#uploader-styling .uploadcare--menu__item,
#uploader-styling .uploadcare--menu__items {
background-color: transparent;
border: 0;
}
#uploader-styling .uploadcare--panel {
flex-direction: column;
}
#uploader-styling .uploadcare--menu {
width: 100%;
height: 60px;
min-height: 60px;
}
#uploader-styling .uploadcare--menu__items {
padding-right: 0;
flex-direction: row;
}
Some dialog elements are rendered as iframe
by Uploadcare servers, which
doesn't let you customize CSS. However, we provide a set of specific methods
to inject CSS into iframes.
Image crop
Cropping images is one of the most common tasks, so we added it right in the jQuery widget UI.
Uploadcare Uploading Widget features a good bunch of crop options, including free
crop. Adding the feature to your Uploading Widget instance is done by implementing
the data-crop
option.
Note that it'll add an additional step of image editing.
How Cropping Works
Technically, image cropping works as post-processing via the Image processing feature:
- Original images go to an Uploadcare project associated with a Public Key set as your Uploading Widget instance.
- The crop is applied as the
crop
image processing operation by injecting its URL directive into original URLs. - The Uploading Widget returns resulting CDN URLs with an injected
crop
.
Configuring Crop
Crop options are held inside the data-crop
attribute as a comma-separated
string with presets names. When you define several presets, users will be able
to choose from the related crop options right in the UI.
Each crop preset is a combination of a size or ratio definition and an optional keyword:
"disabled"
, crop is disabled. It can’t be combined with other presets.""
or"free"
, crop is enabled. Users can freely select any crop area on their images."2:3"
, any area with the aspect ratio of 2:3 can be selected for cropping."300x200"
— same as above, but if the selected area is greater than 300x200 pixels, the resulting image will be downscaled to fit the dimensions."300x200 upscale"
— same as above, but even if the selected area is smaller, the resulting image gets upscaled to fit the dimensions."300x200 minimum"
— users won’t be able to define an area smaller than 300x200 pixels. If an image we apply the crop to is smaller than 300x200 pixels, it will be upscaled to fit the dimensions.
Crop examples
Free crop:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-crop=""
/>
Choosing from predefined aspect ratios:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-crop="free, 16:9, 4:3, 5:4, 1:1"
/>
Fixed aspect ratio:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-crop="4:3"
/>
Fixed size with upscaling:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-crop="400x300 upscale"
/>
Default files in Uploader dialog
Uploadcare Uploading Widget allows you to make specified files appear in the Uploading Widget dialog on open.
Specify these files by adding the value
attribute to your Uploading Widget
<input>
element. The attribute may either be empty or hold a file CDN URL or
UUID.
If you set the value
externally and trigger the DOM change event, it affects
the Uploading Widget. For instance, setting it to a file UUID or a CDN URL will
result in that the file is loaded into the Uploading Widget. You can apply it
anytime, and it'll take effect immediately.
Here’s how you do it:
<input type="hidden" role="uploadcare-uploader" name="my_file"
data-public-key="YOUR_PUBLIC_KEY"
value="https://ucarecdn.com/05da8fb8-bbe9-4da1-a79c-eaf5979152db/"
/>
<!--
Also valid:
value="05da8fb8-bbe9-4da1-a79c-eaf5979152db"
-->
You may also want to check out a live example:
JS snippets and CSS tricks
In this cookbook part, you can find popular code examples and resolutions of common tasks when working with Uploading Widget. Less words, more code!
Paste an image from the clipboard
const widget = uploadcare.Widget("[role=uploadcare-uploader]");
widget.onDialogOpen((dialog) => {
function uploadFromClipboard(e) {
let data = e.clipboardData;
if (!!data && !!data.items.length) {
// check if clipboard data is image
if (data.items[0].type.indexOf("image") != 0) {
alert("No image in the clipboard");
return;
}
let blob = e.clipboardData.items[0].getAsFile();
dialog.addFiles("object", [blob]);
}
}
window.addEventListener("paste", uploadFromClipboard);
});
Get a CDN URL of an uploaded file
For a single-upload widget.
// get a widget reference
const widget = uploadcare.SingleWidget("[role=uploadcare-uploader]");
// listen to the "upload completed" event
widget.onUploadComplete(fileInfo => {
// get a CDN URL from the file info
console.log(fileInfo.cdnUrl);
});
Get CDN URLs of uploaded files
For a multi-upload widget.
// get a widget reference
const widget = uploadcare.MultipleWidget("[role=uploadcare-uploader]");
// listen to the "change" event
widget.onChange(function (group) {
// get a list of file instances
group.files().forEach(file => {
// once each file is uploaded, get its CDN URL from the fileInfo object
file.done(fileInfo => {
console.log(fileInfo.cdnUrl);
});
});
});
Get a group CDN URL
For a multi-upload widget.
// get a widget reference
const widget = uploadcare.MultipleWidget("[role=uploadcare-uploader]");
// listen to the "upload completed" event
widget.onUploadComplete(groupInfo => {
// get CDN URL from group information
console.log(groupInfo.cdnUrl);
});
Get a CDN URL of an uploaded file/group
For a dialog window.
// create a new dialog object
const dialog = uploadcare.openDialog(null, '', {
multiple: false // set to true for multi-file uploads
});
// get a file or group instance
dialog.done(res => {
// once a file or group is uploaded, get its CDN URL
res.promise().done(info => {
console.log(info.cdnUrl);
});
});
Get a camera-recorded video duration
// get widget reference
const widget = uploadcare.Widget("[role=uploadcare-uploader]");
let cameraRecDuration = null;
let recStart = null;
let recEnd = null;
// calculate webcam recording duration
document.body.addEventListener("click", e => {
// user clicks "start recording"
if (e.target.classList.contains("uploadcare--camera__button_type_start-record")) {
recStart = new Date().getTime();
}
// user clicks "stop recording"
if (e.target.classList.contains("uploadcare--camera__button_type_stop-record")) {
recEnd = new Date().getTime();
cameraRecDuration = (recEnd - recStart) / 1000;
console.log("Duration: ", cameraRecDuration);
}
});
Check if a file was added or removed
// get widget reference
const widget = uploadcare.Widget("[role=uploadcare-uploader]")
// get dialog object to access its API
widget.onDialogOpen(dialog => {
// listen to "file added" event
dialog.fileColl.onAdd.add(file => {
console.log("File added", file)
});
// listen to "file removed" event
dialog.fileColl.onRemove.add(file => {
console.log("File removed", file)
});
});
Get the upload source
// get widget reference
const widget = uploadcare.Widget("[role=uploadcare-uploader]");
// listen to the "upload completed" event
widget.onUploadComplete(fileInfo => {
// get source details from file information
console.log(fileInfo.sourceInfo.source);
});
Add an overlay to the crop
.uploadcare--jcrop-tracker::after{
content: "";
position: absolute;
width: 100%;
height: 100%;
left: 0;
/* URL of the overlay image */
background-image: url(<https://i.imgur.com/hSbF6v0.png>);
background-size: cover;
background-position: center;
}
.uploadcare--jcrop-tracker:first-of-type{
opacity: 1 !important;
background-color: transparent !important;
}
Get a selected crop preset
<input type="hidden" role="uploadcare-uploader" name="my_file" id="uploadcare-file" data-crop="2:3, 5:6, 16:9" />
let currentPreset = '2:3';
document.addEventListener('click', e => {
// user clicks on a crop preset icon
if (e.target.classList.contains('uploadcare--crop-sizes__item') || e.target.classList.contains('uploadcare--crop-sizes__icon')) {
// get the caption of the preset selected
currentPreset = document.querySelector('.uploadcare--crop-sizes__item_current').dataset.caption;
console.log(currentPreset);
}
});
Upload an image from Base64
// original base64 image
const b64data = "data:image/gif;base64,R0lGODlhAQABAIAAAP///wAAACH5BAEAAAAALAAAAAABAAEAAAICRAEAOw==";
// convert dataURI to a File object
function dataURLtoFile(dataurl, filename) {
let arr = dataurl.split(','),
mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]),
n = bstr.length,
u8arr = new Uint8Array(n);
while (n--) {
u8arr[n] = bstr.charCodeAt(n);
}
return new File([u8arr], filename, {type:mime});
}
// convert a base64 image to a File object
const fileToUpload = dataURLtoFile(b64data, "image");
// upload the file to Uploadcare
let upload = uploadcare.fileFrom("object", fileToUpload);
upload.done(fileInfo => {
console.log("File uploaded: ", fileInfo.cdnUrl);
});
Render the uploaded image preview
// get widget reference
const widget = uploadcare.Widget("[role=uploadcare-uploader]");
widget.onUploadComplete(fileInfo => {
const preview = document.createElement("img");
// create a preview of the uploaded image of 200px width
preview.src = fileInfo.cdnUrl + "-/resize/200x/";
// add the thumbnail to the page
document.body.appendChild(preview);
});
Add custom names to crop presets
<input type="hidden" role="uploadcare-uploader" name="my_file" id="uploadcare-file" data-crop="free,1:1,2:3" />
.uploadcare--crop-sizes__item {
overflow: visible;
}
.uploadcare--crop-sizes__item:nth-child(1)::after {
content: "Free form";
}
.uploadcare--crop-sizes__item:nth-child(2)::after {
content: "Square";
}
.uploadcare--crop-sizes__item:nth-child(3)::after {
content: "Portrait";
}
Add custom widget button labels
<input type="hidden" role="uploadcare-uploader" id="uploadcare-file-1" data-btn-text="I'm the first buton" />
<input type="hidden" role="uploadcare-uploader" id="uploadcare-file-2" data-btn-text="I'm the second one" />
<input type="hidden" role="uploadcare-uploader" id="uploadcare-file-3" data-btn-text="And I am the third" />
// get an array of widget references
const widgets = uploadcare.initialize();
// update each widget button text with the data-btn-text attribute's value
widgets.forEach(widget => {
widget.inputElement.nextSibling.querySelector(".uploadcare--widget__button_type_open").innerHTML = widget.inputElement.getAttribute("data-btn-text");
});
Reset widget after upload
// get widget reference
const widget = uploadcare.Widget("[role=uploadcare-uploader]");
// once a file is uploaded, output its URL and reset the widget
widget.onUploadComplete(fileInfo => {
console.log(fileInfo.cdnUrl);
widget.value(null);
});
Add a custom message to the dialog
/* turn the "after" pseudo element of the dialog container's div into a hint */
.uploadcare--tab__content::after {
content: "Wake up Neo... The Matrix has you... Follow the white rabbit🐇";
padding: 1em;
margin: 2em;
background-color: #000;
color: #00ff00;
border-radius: 5px;
}
Video record time limit
const SECOND = 1000;
// record time limit
const TIME_LIMIT = 5 * SECOND;
const widget = uploadcare.Widget("[role=uploadcare-uploader]");
widget.onDialogOpen((dialog) => {
// Listen to a click on the Start button
dialog.dialogElement[0].addEventListener("click", (e) => {
if (
e.target.classList.contains(
"uploadcare--camera__button_type_start-record"
)
) {
const stopBtn = dialog.dialogElement[0].querySelector(
".uploadcare--camera__button_type_stop-record"
);
// Diasplay countdown on Stop button
let remaining = TIME_LIMIT;
stopBtn.innerText = `Stop (in ${remaining / 1000}s)`;
let counter = setInterval(() => {
remaining -= 1000;
if (remaining <= 0) {
clearInterval(counter);
}
stopBtn.innerText = `Stop (${remaining / 1000}s)`;
}, 1000);
// Click Stop button (stop recording) after TIME_LIMIT
setTimeout(() => {
stopBtn.click();
}, TIME_LIMIT);
}
});
});
Versioning
When we introduce backward-incompatible changes, we release new major versions. Once published, such versions are supported for 2 years. You will still be able to use any file uploader version after its support term at your own risk.
Version | Date Published | Supported Until |
---|---|---|
3.x | 28 Jun 2017 | TBD |
2.x | 20 Feb 2015 | 1 Jan 2020 |
1.x | 21 Mar 2014 | 1 Jun 2019 |
0.x | 6 Sep 2012 | 1 Jun 2019 |
We're building the next uploader! Check out new File uploader.