Image optimization for Next.js applications
In this article, we will explore various methods of optimizing images in Next.js applications, as well as the benefits of using Uploadcare’s Next.js image loader and component over other solutions.
Next.js Image
component
Next.js offers a built-in image component that extends the regular <img>
element
to help you optimize images on-demand when images are being served to your users.
The Next.js Image
component is designed to improve the performance of your website
by automatically optimizing images and serving them in the most efficient way possible.
This image component creates a list of srcset
of image sizes
and automatically selects the appropriate size for users based on their current browser viewport width.
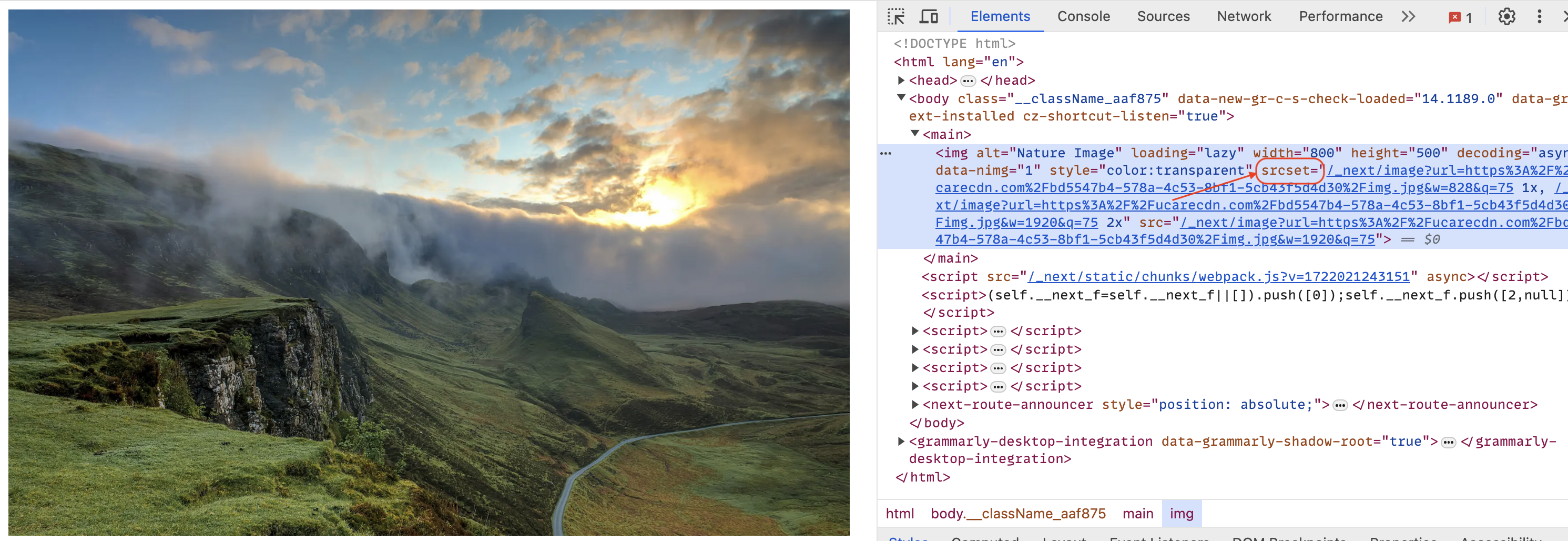
Using Vercel’s Image Optimization API,
the Image
component generates different image sizes and formats to serve to users.
The API also caches images generated by and serve them via Vercel’s CDN so your users can quickly access them.
By default, for every new Next.js application created, the Next.js image component is installed. To use it in your application, import it into your component using the code:
import Image from 'next/image';
You can then use in your components:
<Image
src={image}
alt="nature image"
width={800}
height={600}
/>
Features of Next.js Image
component
Some key features of the Image
component by Next.js include:
Automatic Image Optimization
-
The image component automatically generates different versions for different device screen sizes and pixel ratios.
-
Next.js image component serves images in modern formats like WebP or AVIF when supported by the browser, ensuring efficient delivery and reduced file sizes.
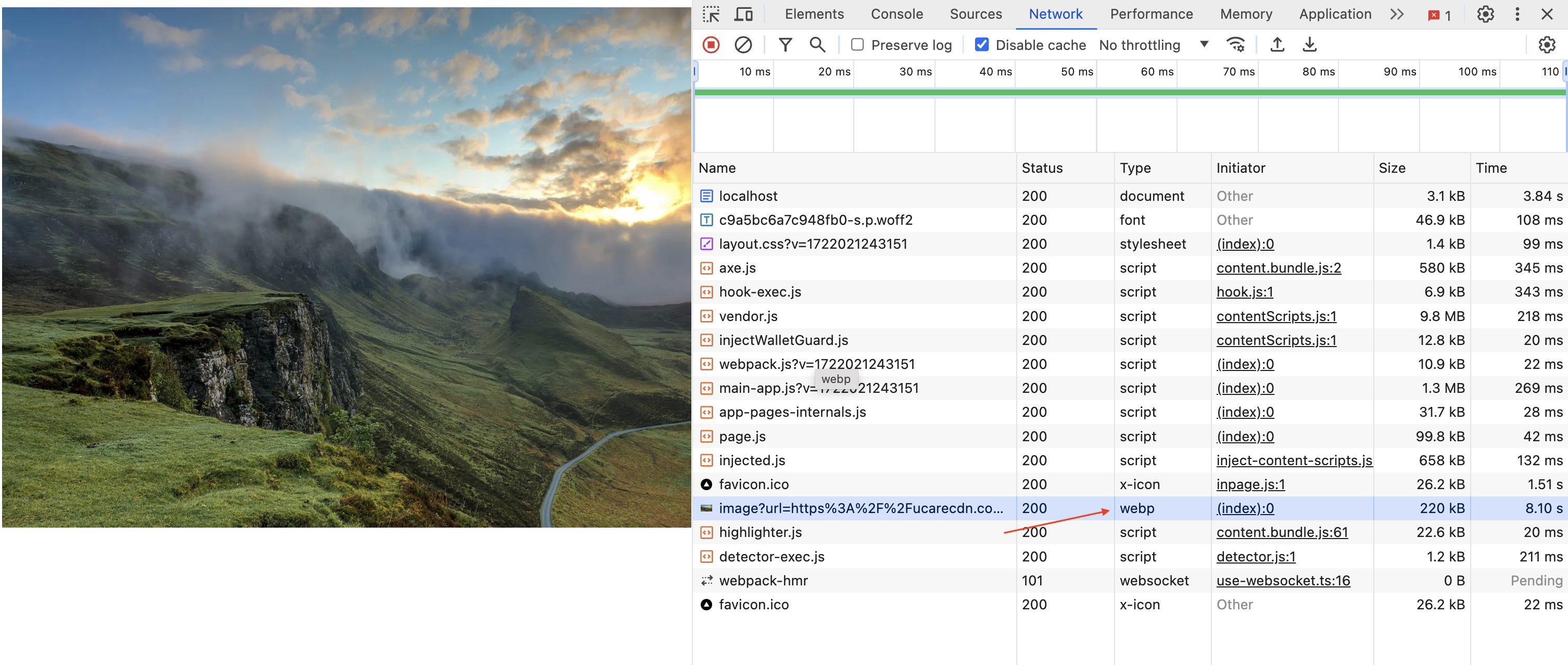
Lazy Loading
The component supports lazy loading of images and loads images only when they enter the viewport, thereby reducing initial page load time.
To use this feature, set the loading props to be lazy:
<Image
loading="lazy"
src={image}
alt="nature image"
width={800}
height={600}
/>
Priority Loading
Priority loading is a feature that ensures critical images are loaded as soon as possible to improve your web page’s Largest Contentful Paint (LCP) and overall page performance. This is particularly useful for images visible above the fold when a page first loads.
The priority
attribute can be added to the Image
component.
Images with priority
are preloaded, meaning they are fetched earlier than other images, ensuring faster rendering.
To prioritize a specific image on a page, add the priority
props to the component:
<Image
src={img}
alt="nature image"
priority
width={800}
height={600}
/>
For a complete list of the features and customization options available with the Next.js Image
component,
visit the official
Next.js image optimization documentation.
Tips and best practices for image optimization when using Next.js image component
When using the Next.js image component, consider the following tips and best practices to ensure optimal performance and user experience:
-
Use
alt
props: Always include descriptivealt
text for images. This improves accessibility for screen readers and enhances SEO by providing context to search engines. -
Specify
height
andwidth
for remote images: Defining explicit height and width attributes helps Next.js calculate aspect ratios, preventing Cumulative Layout Shifts (CLS) and improving performance. -
Use the
priority
prop for high-priority images: Apply the priority attribute to critical images that should load first. This ensures essential visuals are displayed quickly, improving user experience and Largest Contentful Paint (LCP). -
Use static imports for local images: Importing local images statically allows Next.js to optimize them automatically during build time, resulting in smaller file sizes and faster load times.
Uploadcare Next.js loader for images in Next.js applications
Uploadcare enhances the image experience in your Next.js applications by providing a robust image optimization solution that can be used in any Next.js application, nextjs-loader.
The component uses Uploadcare’s optimization and transformation API to optimize images in your Next.js application. Then it caches these images in the Uploadcare CDN to enable us to serve these images to your users as fast as possible.
The nextjs-loader library provides an UploadcareImage
component that functions identical
to the Next.js Image
component but with some extra optimization features.
The UploadcareImage
component also supports all the props available in the Next.js Image
component,
such as src
, alt
, width
, height
, priority
, etc.
To use the component and loader, install it in your application with the command:
npm install @uploadcare/nextjs-loader
Update the next.config.mjs file to load images from the Uploadcare CDN:
/** @type {import('next').NextConfig} */
const nextConfig = {
images: {
remotePatterns: [
{
protocol: 'https',
hostname: 'ucarecdn.com',
port: '',
pathname: '/**',
},
],
},
};
export default nextConfig;
Next, create a .env.local file and add your Public key from your Uploadcare project dashboard:
#.env.local
NEXT_PUBLIC_UPLOADCARE_PUBLIC_KEY="YOUR_PUBLIC_KEY"
The image component also supports transformation operations provided by the Uploadcare operations API that can be used to load better-optimized images.
To use these transformations, update your env.local file with the values:
#.env.local
NEXT_PUBLIC_UPLOADCARE_TRANSFORMATION_PARAMETERS="format/auto, stretch/off, progressive/yes, quality/lightest"
Where:
-
format
: is the format of the image to be served. This could be specified asjpeg
,png
, orwebp
image format; when set toauto
, the image component determines the best image format to serve based on what image format a client browser supports. -
progressive
: tells Uploadcare to loadjpeg
images in a progressive manner, which improves the loading experience by displaying a lower-quality version of the image initially, and gradually enhancing it as more data is downloaded. -
quality
: tells Uploadcare to optimize the image to the smallest file size possible with the highest compression ratio while maintaining acceptable quality. This results in approximately 50% of the original file size.
Check out the transformation documentation for a list of transformation operations you can apply to images.
To use the UploadcareImage
in your component, import the UploadcareImage
component and render with the src value as the URL of your image:
import UploadcareImage from '@uploadcare/nextjs-loader';
<UploadcareImage
alt="A test image"
src="https://ucarecdn.com/bd5547b4-578a-4c53-8bf1-5cb43f5d4d30/img.jpg"
width="800"
height="500"
/>
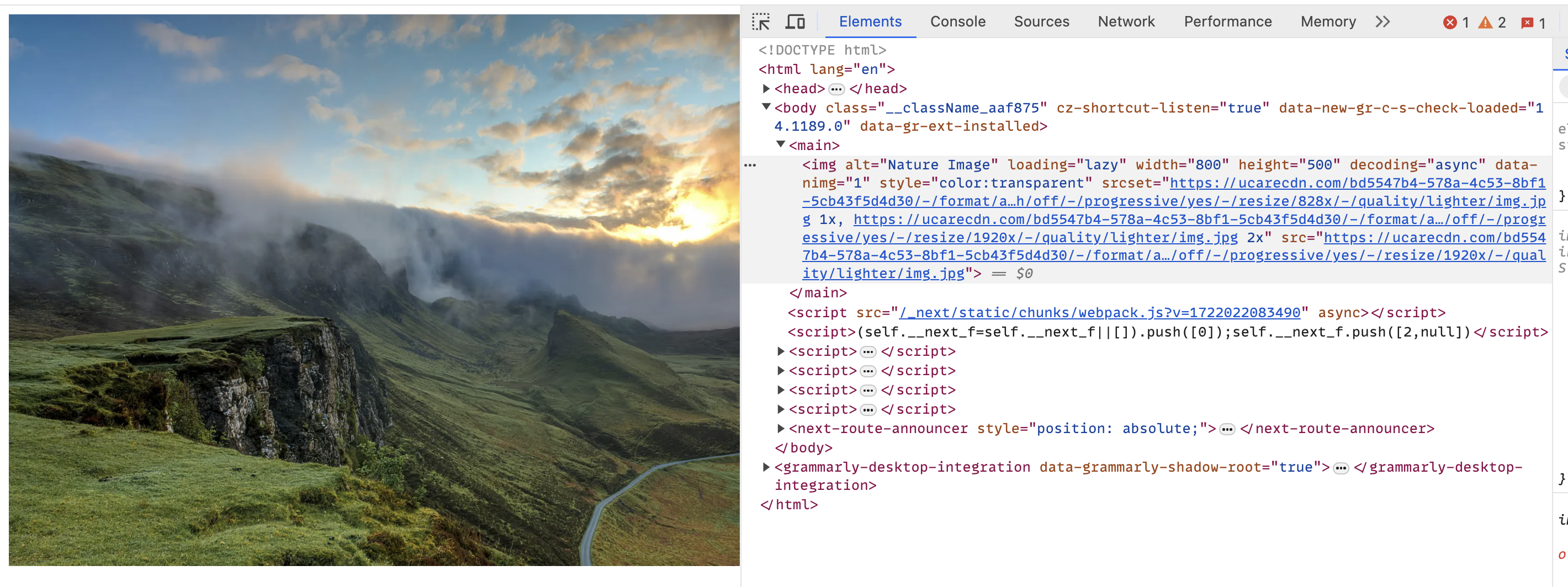
The nextjs-loader library also provides a loader that can be used along with the Next.js Image
component. This loader can be used to load images from the Uploadcare CDN and apply transformations to images on the fly.
To use the loader, update your next.config.mjs file to use the loader from the nextjs-loader library:
/** @type {import('next').NextConfig} */
const nextConfig = {
images: {
// previous image configurations
loader: 'custom',
},
};
export default nextConfig;
You can now import the Uploadcare image loader from nextjs-loader to use in your Image components:
import { uploadcareLoader } from '@uploadcare/nextjs-loader';
<Image
src="https://ucarecdn.com/bd5547b4-578a-4c53-8bf1-5cb43f5d4d30/img.jpg"
alt="Nature Image"
width={800}
height={500}
loader={uploadcareLoader}
/>
If you’d like to use uploadcareLoader to optimize all images in your Next.js application,
update next.config.mjs to load the uploadcareLoader
as a loader file:
/** @type {import('next').NextConfig} */
const nextConfig = {
images: {
// previous image configurations
loader: 'custom',
loaderFile: './node_modules/@uploadcare/nextjs-loader/build/loader.js',
},
};
export default nextConfig;
You can now use the Next.js Image component without specifying loader props, and Uploadcare will handle all image optimization for you:
<Image
src="https://ucarecdn.com/bd5547b4-578a-4c53-8bf1-5cb43f5d4d30/img.jpg"
alt="Nature Image"
width={800}
height={500}
/>
For images not uploaded to Uploadcare servers, such as local images, the nextjs-loader library optimizes such images via a proxy. To use the proxy, update your env.local file to include your application’s URL as the base URL for the proxy:
NEXT_PUBLIC_UPLOADCARE_APP_BASE_URL="https://your-domain.com/"
In your Project -> Settings -> Delivery, add your domain URL to the list of allowed domains for proxy.
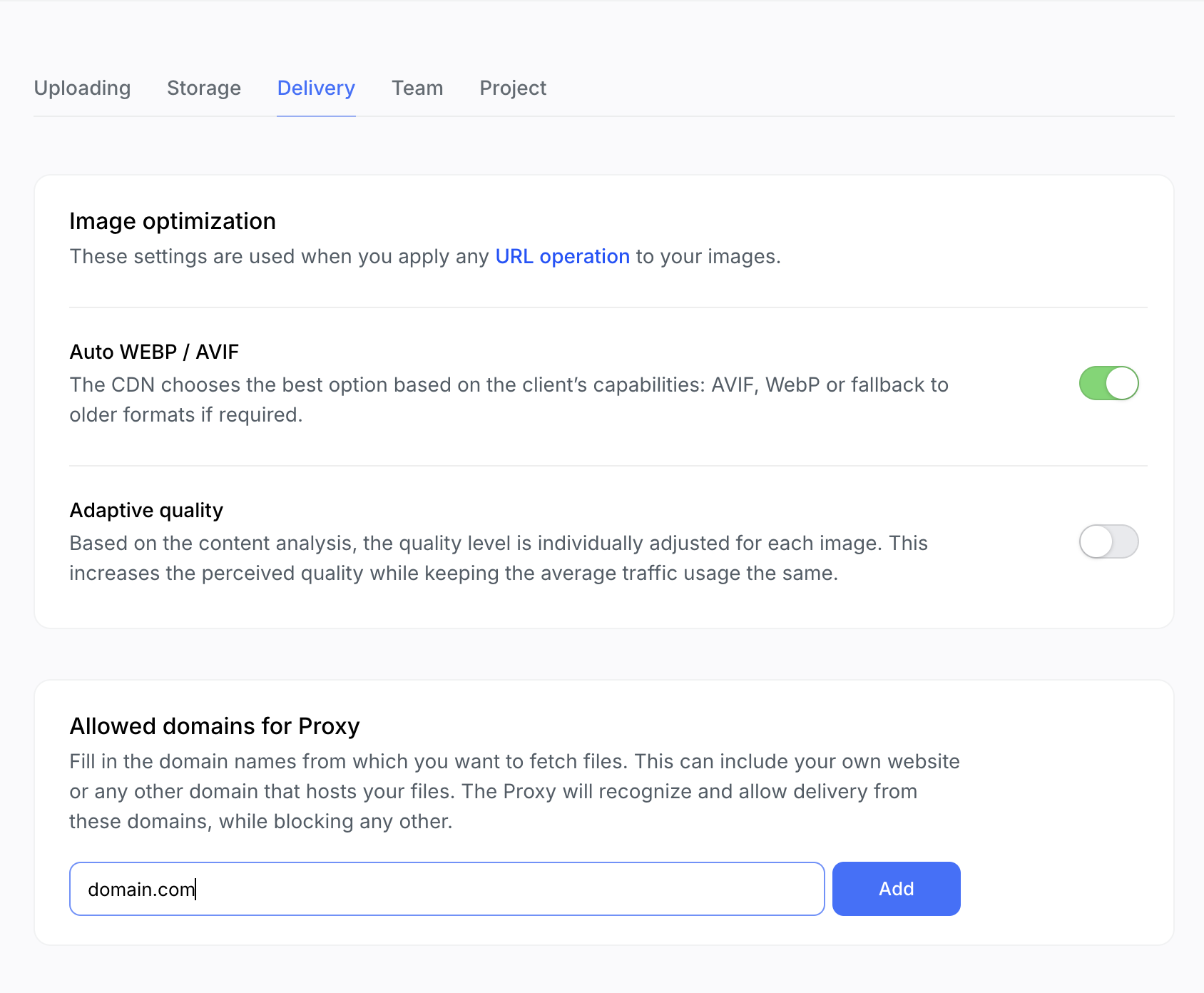
You can now use the UploadcareImage
component with local images on your website:
<UploadcareImage
src={img}
alt="Nature Image"
width={800}
height={500}
/>
Note: Local images in development will not be optimized but will be served as they are; however, when your project is deployed to a different environment, Uploadcare CDN will optimize all images via proxy.
Benefits of using Uploadcare image component and loader
-
Uploadcare transformations: Using the
UploadcareImage
component, you can apply different transformation operations like resizing, cropping, and format conversion. These transformations are applied on the fly, ensuring images are optimized for any use case without manual intervention. -
Responsive images: The
UploadcareImage
component automatically generates images tailored to different devices and screen sizes. This ensures the best quality and performance by delivering the optimal image for each user’s device. -
Uploadcare CDN for fast delivery: The component leverages Uploadcare’s global CDN to deliver images quickly and efficiently. This minimizes latency and ensures fast load times for users worldwide, improving overall user experience.
-
Proxy images: Utilize Uploadcare’s proxy functionality to serve external images seamlessly. This feature fetches images from any URL and applies optimizations such as resizing and real-time format conversion. By caching and serving images through Uploadcare’s CDN, you ensure consistent performance and security, reducing load times and enhancing user experience.
Conclusion
Image optimization is vital to enhancing the performance of Next.js applications.
Using the built-in Next.js Image component, you can automatically optimize images for different devices and browsers,
improving load times and user experience.
Additionally, by integrating Uploadcare’s solutions, such as the UploadcareImage
component and loader,
you can further enhance image delivery with advanced transformations and a robust CDN.