How to generate PDF thumbnails for the web using Uploadcare
Ever wondered how to give your users a sneak peek of a PDF before they open it? PDF thumbnails are the answer! Thumbnails are small images that represent the first page of a PDF document.
In this article, you will learn how to generate a thumbnail image for your PDF documents using Uploadcare’s document converter API. We cover how to create the image thumbnail and listen for the document conversion completion using webhooks to get the thumbnail URL.
The document converter also allows you to convert various document formats to another, but for this article, we will focus only on generating a thumbnail for a PDF document.
Ready? Let’s dive in! 🚀
Why thumbnails matter
Before jumping into the technical details, let’s talk about why having a thumbnail matters.
Thumbnails give your users a visual preview, helping them identify content faster. They’re perfect for improving user experience, whether in a document library, a CMS, or any platform handling PDFs.
How to generate a thumbnail for a PDF document using Uploadcare
You can generate a thumbnail using Uploadcare’s Rest API document converter endpoint. The endpoint allows you to convert a document, such as a PDF, to a different document, such as Microsoft Docs or even an image.
Conversion happens in two ways: first, you send a request to the document conversion endpoint along with the file URL you want to convert and the format (for conversion to images) or document you want to convert to.
The API then returns the new file’s UUID and a token that you can use to confirm whether the conversion process has been completed or is still pending or processed.
Lastly, you can then choose to either use the Uploadcare webhooks to listen for changes to the document or send an API request to get the newly generated file.
Let’s see this in action, shall we?
Note: The Rest API is also available as SDKs in various languages such as JavaScript, PHP, Python, Ruby, Swift, and Kotlin. For their Implementation, please visit the API documentation.
Using the Uploadcare Rest API document converter
First, let’s send a request to the document conversion API endpoint:
// Replace these constants with your data
const YOUR_PUBLIC_KEY = 'your_public_key';
const YOUR_SECRET_KEY = 'your_secret_key';
const UUID = 'your_file_uuid';
const generatePath = (UUID) => `https://ucarecdn.com/${UUID}/`;
const requestBody = {
paths: [`${generatePath(UUID)}document/-/format/jpg/-/page/1/`],
store: '1',
};
fetch('https://api.uploadcare.com/convert/document/', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
Accept: 'application/vnd.uploadcare-v0.7+json',
Authorization: `Uploadcare.Simple ${YOUR_PUBLIC_KEY}:${YOUR_SECRET_KEY}`,
},
body: JSON.stringify(requestBody),
})
.then((response) => response.json())
.then((data) => {
const token = data.result[0].token;
const image = generatePath(data.result[0].uuid);
console.log({ token, image })
})
.catch((error) => {
console.error('Error:', error);
});
The code above performs the following:
-
Creates a
paths
array, which contain the URLs of the files you want to convert and the format you want to convert to, in this case, to ajpg
image format, and only a single page (page 1) should be converted. -
Passes a
store
value of1
indicating that when the image is generated, it should be stored in the project -
Sends a request to the endpoint using the
paths
array andstore
value as the body of the request -
When the server gets the request, it converts the first page of the PDF file to an image and returns an object with two values:
problems
andresult
.
Inside the result
array, you’ll find the uuid
of the newly generated image and a token
that you can use to confirm whether the conversion process has been completed or is still pending or processing.
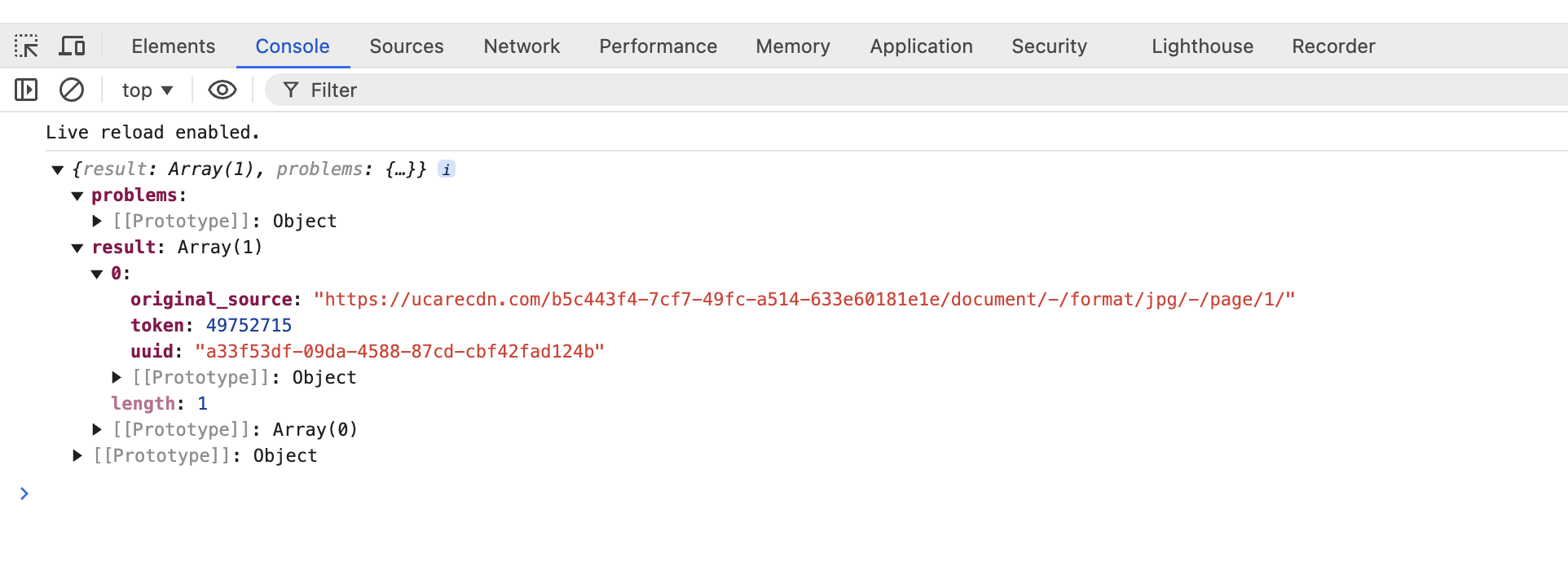
Using the token
, you can check the status of the conversion process
by sending a request to the document conversion status endpoint with the token
as a query parameter.
fetch(`https://api.uploadcare.com/convert/document/status/${token}/`, {
headers: {
'Content-Type': 'application/json',
Accept: 'application/vnd.uploadcare-v0.7+json',
Authorization: `Uploadcare.Simple ${YOUR_PUBLIC_KEY}:${YOUR_SECRET_KEY}`,
},
})
.then((response) => response.json())
.then((data) => {
console.log('Response:', data);
})
.catch((error) => {
console.error('Error:', error);
});
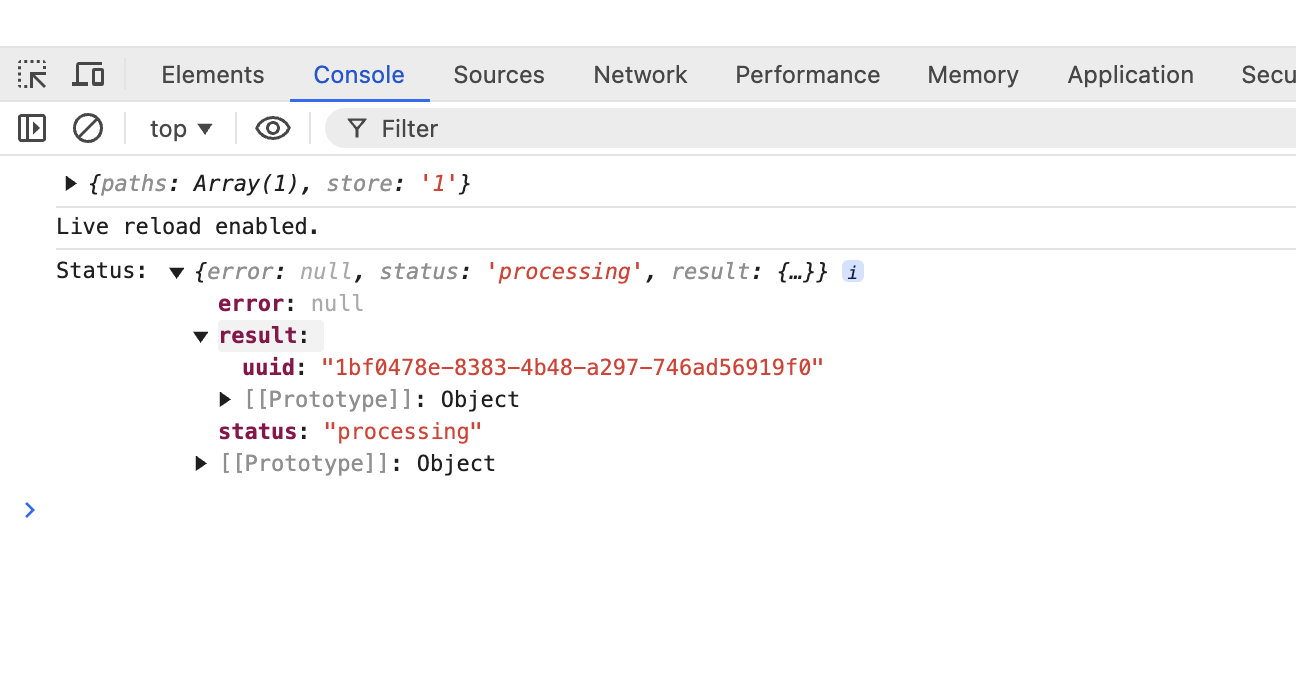
The status endpoint returns an object with the following fields:
-
error
: for any errors encountered during the conversion process -
result
: An object that contains the UUID of the newly generated file -
status
: The status of the conversion process, which can bepending
,processing
,finished
,failed
, orcancelled
If the status is finished
, you can use the newly generated image’s uuid
to display the thumbnail on your website.
There is a better way to check if the file conversion process is completed automatically—webhooks! Let’s look at this in the next section of this article.
Listening for the document conversion completion using webhooks
Uploadcare has a set of webhooks that you can use to listen to changes relating to the files in your project.
You can use these webhooks to listen for changes to the document conversion process and get the URL of the newly generated image.
An example of the perfect webhook you could use to check
if the thumbnail of your document has been generated is the file.uploaded
webhook,
which is used when a new file has been uploaded.
The webhook then sends a POST request to the URL you specify, containing the details of the file that has been uploaded and some information on who triggered the webhook.
Let’s create a new webhook that listens for the file.uploaded
event
and gets a response from the webhook when the document conversion process is completed.
To use a webhook, you must first enable it on your project from the Webhooks Settings page. Then, click the “Add Webhook” button to add a new webhook.
This will open up a modal to fill in the details for the webhook you want to create:
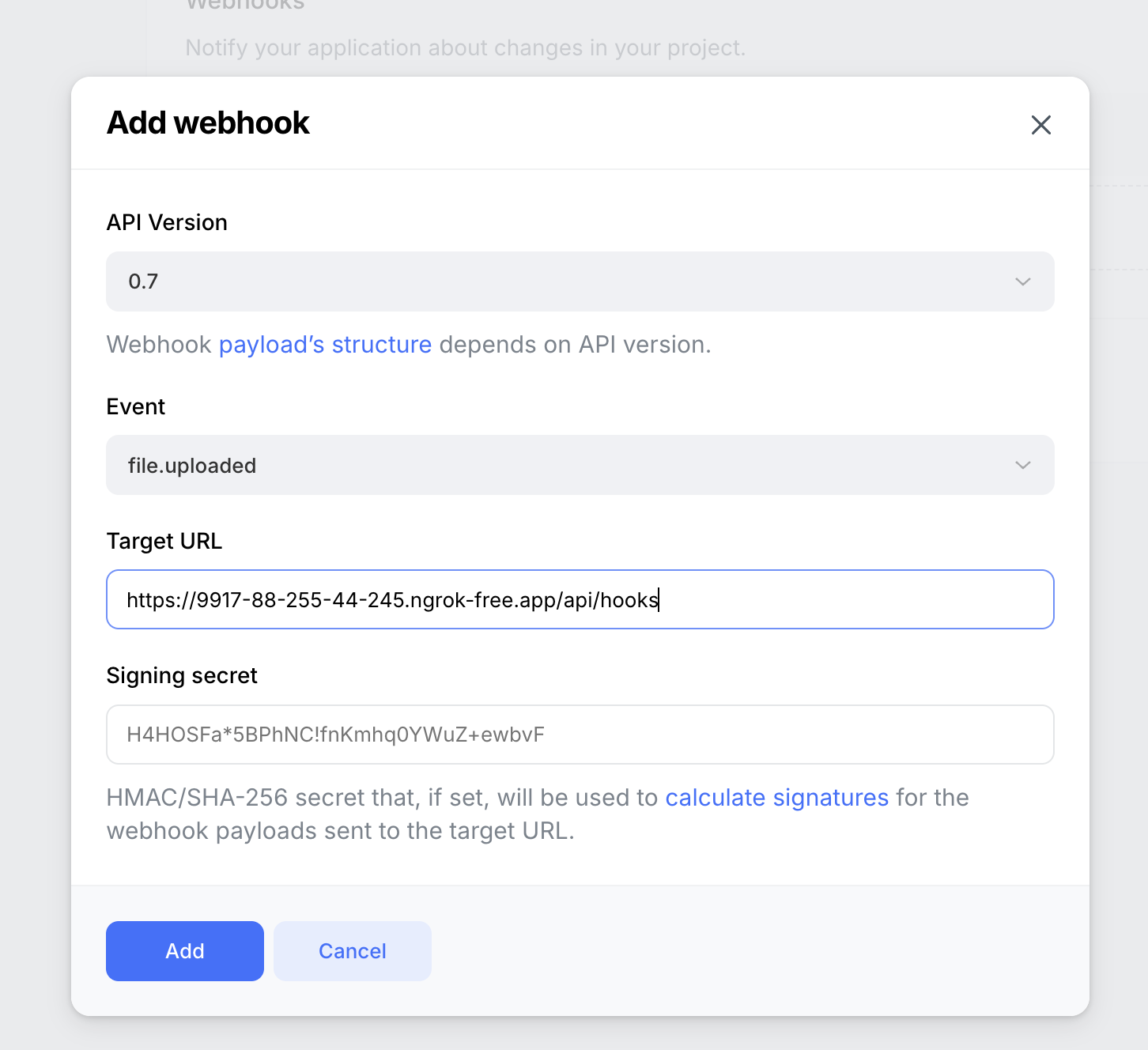
Where:
-
Event should be
file.uploaded
to listen for updated changes. -
The target URL should be the URL where you want to listen for the hook event.
-
Signing secret is a unique secret hash generated by you for extra security when Uploadcare interacts with your server.
When a new file is uploaded into the project, the hook will return data about the file like this:
{
"initiator": {
"type": "addon",
"detail": {
"addon_name": "zamzar_convert_document",
"request_id": "8ccfedfd-f975-4f1c-bbfa-41e9fa500d3c",
"source_file_uuid": "7b9811fa-f4ca-4382-a6c8-1716f755f17a"
}
},
"hook": {
"id": 2111883,
"project_id": 150884,
"project_pub_key": "83700ef90120003b1469",
"target": "https://9917-88-255-44-245.ngrok-free.app/api/hooks",
"event": "file.uploaded",
"is_active": true,
"version": "0.7",
"created_at": "2025-01-14T08:57:06.172377Z",
"updated_at": "2025-01-14T09:12:44.835682Z"
},
"data": {
"content_info": {
"mime": {
"mime": "image/jpeg",
"type": "image",
"subtype": "jpeg"
},
"image": {
"color_mode": "RGB",
"format": "JPEG",
"height": 3375,
"width": 6000,
"orientation": null,
"dpi": null,
"geo_location": null,
"datetime_original": null,
"sequence": false
}
},
"uuid": "088f5961-c90e-4e8c-a031-cf65b51896db",
"is_image": true,
"is_ready": true,
"metadata": {},
"appdata": {
"uc_clamav_virus_scan": {
"data": {
"infected": false
},
"version": "1.0.1",
"datetime_created": "2025-01-14T12:08:22.424Z",
"datetime_updated": "2025-01-14T12:08:22.425Z"
}
},
"mime_type": "image/jpeg",
"original_filename": "sample_compressed-0.jpg",
"original_file_url": "https://ucarecdn.com/088f5961-c90e-4e8c-a031-cf65b51896db/sample_compressed0.jpg",
"datetime_removed": null,
"size": 938994,
"datetime_stored": "2025-01-14T12:08:22.37374Z",
"url": "https://api.uploadcare.com/files/088f5961-c90e-4e8c-a031-cf65b51896db/",
"datetime_uploaded": "2025-01-14T12:07:42.609637Z",
"variations": null
},
"file": "https://ucarecdn.com/088f5961-c90e-4e8c-a031-cf65b51896db/sample_compressed0.jpg"
}
Notice the initiator of the hook is the document converter API;
this is because the document conversion process was triggered by the document converter API.
Also the object contains a file
field that contains the URL of the newly generated image.
With this data, you can then use the URL of the newly generated image to display the PDF thumbnail on your website.
Conclusion
In this article, you learned how to generate a thumbnail for your PDF documents using Uploadcare’s document converter API. You created the image thumbnail and used webhooks to listen for the document conversion completion to get the thumbnail URL.
Aside from making generating thumbnails easier, Uploadcare also provides you with other features you can perform with documents, such as converting a document (e.g. PDF or DOC) to another document format (e.g. CSV or EPUB) and a File Uploader for uploading documents from your applications.