File uploads with HTML
Last edited:
It is quite common for websites or apps to allow a user to upload files as a feature or part of a feature. For example, HTML file uploads could be used to allow users to upload avatars, or allow an internal team to upload photos of products to a website or app. In this tutorial we will briefly look at file uploads, and how to set this up in your coding. This tutorial assumes some knowledge and understanding of coding and web development. This post is meant as a brief overview. Let’s get into it!
<input type="file">
Luckily for us, HTML provides a fairly simple solution which enables us to upload files, the <input>
element!
Taking a look at this, a limited example of how we’d code an upload file button in HTML could look like this:
<label for="photo">Choose a photo!</label>
<input
type="file"
id="photo"
name="photo"
accept="image/*">
You should see the following if you run an HTML page on a localhost server:

Clicking on the Choose File button should bring up your Operating System’s file selection option.
If we wanted to customize the text within the button to something other than Choose File we could do something like:
<span>
File Upload
<input
type="file"
id="photo"
name="photo"
accept="image/png, image/jpeg">
</span>
That gets us the button and the ability to choose the file. How would we direct the file to our server once it’s selected? To direct the file, we would make the button part of a form which would then activate a Script (could be JavaScript, PHP, etc). The logic in this Script would then tell the server what to do with the file once it’s uploaded. We won’t go over those kinds of Scripts in this post. However, the code to link to the Script would look something like this:
<form action="yourScript">
<input type="file" id="myFile" name="filename">
<input type="submit">
</form>
Hiding the button
In some instances, you may want to hide a file upload button. The way to do this typically relies on CSS.
This is one way to do it, we could attach the CSS to our input and do the following:
input {
opacity: 0;
z-index: -1;
position: absolute;
}
opacity: 0
— makes the input transparent.z-index: −1
— makes sure the element stays underneath anything else on the page.position: absolute
- make sure that the element doesn’t interfere with sibling elements.
We would set this as the default CSS, then we would write a short script that would change the CSS once someone selected a file, so that the user could see a Submit button, for instance.
There are a couple of other potential CSS options:
input {
display: none;
}
And:
input {
visibility: hidden;
}
These options should be avoided, as they do not work well with accessibility readers. opacity: 0
is the preferred method.
Further customization
There is a very good chance that we would want to change the look of our file upload buttons from the rather ugly grey default buttons to something a bit more pleasing to the eye.
As one would guess, button customization involves CSS.
We know that we can put the input in the <span></span>
tags to customize the text that appears on the button.
Another method is the <label></label>
tags.
Let’s try this!
<input type="file" name="file" id="file" class="myclass" />
<label for="file">Choose a file</label>
.myclass + label {
font-size: 2em;
font-weight: 700;
color: white;
background-color: green;
border-radius: 10px;
display: inline-block;
}
.myclass:focus + label,
.myclass + label:hover {
background-color: purple;
}
This will get us a green button that will turn purple when we hover the mouse cursor over it, it should look like this:

However, we are now presented with a problem! How do we get rid of the default input option on the left (since we would only want the one custom button)? Remember how we learned how to hide buttons earlier? We can put that into practice now.
Add the following CSS to the previous CSS code:
.myclass {
width: 0.1px;
height: 0.1px;
opacity: 0;
overflow: hidden;
position: absolute;
z-index: -1;
}
Boom! Problem solved:
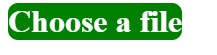
Getting information on files
There may be instances in which we want to gather information about files which the user is uploading to our server. Every file includes file metadata, which can be read once the user uploads said file(s). File metadata can include things such as the file’s MIME type (what kind of media it is), file name, size, date the file was last modified... Let’s take a quick look at how we could pull up file metadata — this will involve some JavaScript.
<input type="file" multiple onchange="showType(this)">
function showType(fileInput) {
const files = fileInput.files;
for (let i = 0; i < files.length; i++) {
const name = files[i].name;
const type = files[i].type;
alert('Filename: ' + name + ' , Type: ' + type);
}
}
If we run this code, we will see a Choose File button. When we choose a file from our device, a browser popup box will appear. The browser popup will inform us of the filename and file type. Of course there is logic that we can write to change the type of file metadata that you gather, and what happens with it, depending on our needs.
Limiting accepted file types
We, of course, can think of many instances where one would want to limit which kinds of files the user can choose to upload to your server (security considerations among the many reasons to consider).
Limiting accepted file types is quite easy — to do this we make use of the accept attribute within the <input>
element.
An example of how we would do this is:
<input
type="file"
id="photo"
name="photo"
accept=".jpg,.jpeg,.png">
We can specify which specific file formats you want to accept, like we did above, or we can simply do:
<!-- other attributes are omitted for simplicity -->
<input accept="image/*">
There are ways you can limit formats and file types for other types of files as well, but we won’t cover everything here.
The Uploadcare uploader
Uploadcare features a handy File Uploader feature. The Uploadcare File Uploader is responsive, mobile-ready, and easy to implement. For full details on our File Uploader please visit /docs/uploads/file-uploader/
To use the Uploadcare File Uploader, you would need to import the File Uploader library in your HTML:
<script type="module">
import * as UC from 'https://cdn.jsdelivr.net/npm/@uploadcare/file-uploader@v1/web/file-uploader.min.js';
UC.defineComponents(UC);
</script>
<link
rel="stylesheet"
href="https://cdn.jsdelivr.net/npm/@uploadcare/file-uploader@v1/web/uc-file-uploader-regular.min.css"
>
And in the <body>
of your HTML, you would need to add the following code:
<uc-config
ctx-name="my-uploader"
pubkey="YOUR_PUBLIC_KEY"
>
</uc-config>
<uc-file-uploader-regular ctx-name="my-uploader"></uc-file-uploader-regular>
Where YOUR_PUBLIC_KEY
is your Uploadcare Public Key from API Keys
in your Uploadcare dashboard.
The <uc-config>
element configures the uploader.
It uses the ctx-name
attribute to identify the uploader and the pubkey
attribute to specify your Uploadcare Public Key.
The <uc-file-uploader-regular>
element creates the uploader.
The ctx-name
attribute specifies the uploader to which the configuration applies.
And there you have it! That was a brief overview on the basics of uploading files to a server using HTML. Now get out there and try implementing what we’ve learned in a project!