File uploader validation guide
Using file validators allows you to apply specific restrictions during file uploads to ensure quality and consistency. These validations can occur before, during, or after the upload process. In this article, we will consider the different validators that come with our File Uploader and create a couple of validators f or uploading files in an e-commerce store that sells furniture. By utilizing these validators, you can guarantee that all images of products meet the necessary dimensions, file size, and consistency for the e-commerce store.
To begin implementing the validators for the e-commerce images, let’s first consider the requirements that the images uploaded for the project must meet:
- Maximum file size: Limit the maximum file size to 2 MB to keep load times fast and improve user experience.
- Uniform dimensions: Ensure all images are 1080×1080 pixels, thus have a 1:1 aspect ratio and allow clear, high-quality zooming.
- Collection limit: Each collection of images for a single product must have 3 images, a front, back, and side view, to provide a comprehensive view of the product.
Project setup
Let’s set up the File Uploader in a project to implement the validators. You can add the file uploader to your HTML page using the following code snippet.
First, import the File Uploader components:
<script type="module">
import * as UC from 'https://cdn.jsdelivr.net/npm/@uploadcare/file-uploader@v1/web/file-uploader.min.js';
UC.defineComponents(UC);
</script>
Next, import the File Uploader CSS:
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@uploadcare/file-uploader@v1/web/uc-file-uploader-regular.min.css">
Finally, add the File Uploader components to your HTML page:
<uc-config
ctx-name="my-uploader"
pubkey="YOUR_PUBLIC_KEY"
></uc-config>
<uc-file-uploader-regular
ctx-name="my-uploader"
></uc-file-uploader-regular>
Replace YOUR_PUBLIC_KEY
with your Uploadcare public key.
This key is required to authenticate your requests when uploading files using the File Uploader.
Types of validators
There are two types of validators that the File Uploader provides to ensure that uploaded files meet the required criteria: Built-in validators and Custom validators. Each type of validator serves a specific purpose and can be used to enforce different validation rules based on your project requirements.
1. Built-in validators
Built-in validators are predefined within the File Uploader and cover the basic, commonly used validation checks needed for your project requirements. Some of the built-in validators include:
- max-local-file-size-bytes: Limits file size to a specified maximum. This helps optimize load times, particularly on e-commerce platforms.
- img-only: Restricts uploads to only allow images to be uploaded. This ensures that only images are uploaded to the e-commerce platform.
For the e-commerce project, let’s use the built-in validators to enforce a file size limit of 2 MB and only allow images to be uploaded.
To do this, update the <uc-config>
component to use the max-local-file-size-bytes
and img-only
attributes:
<uc-config
ctx-name="my-uploader"
pubkey="YOUR_PUBLIC_KEY"
max-local-file-size-bytes="2000000"
img-only
></uc-config>
With the above configuration, the File Uploader will only allow image files to be uploaded and will restrict the file size to a maximum of 2 MB. This ensures that all product images meet the required quality standards for the e-commerce platform.
If an uploaded file does not meet the specified requirements, the File Uploader will display an error message to the user, stating that the file is too big.
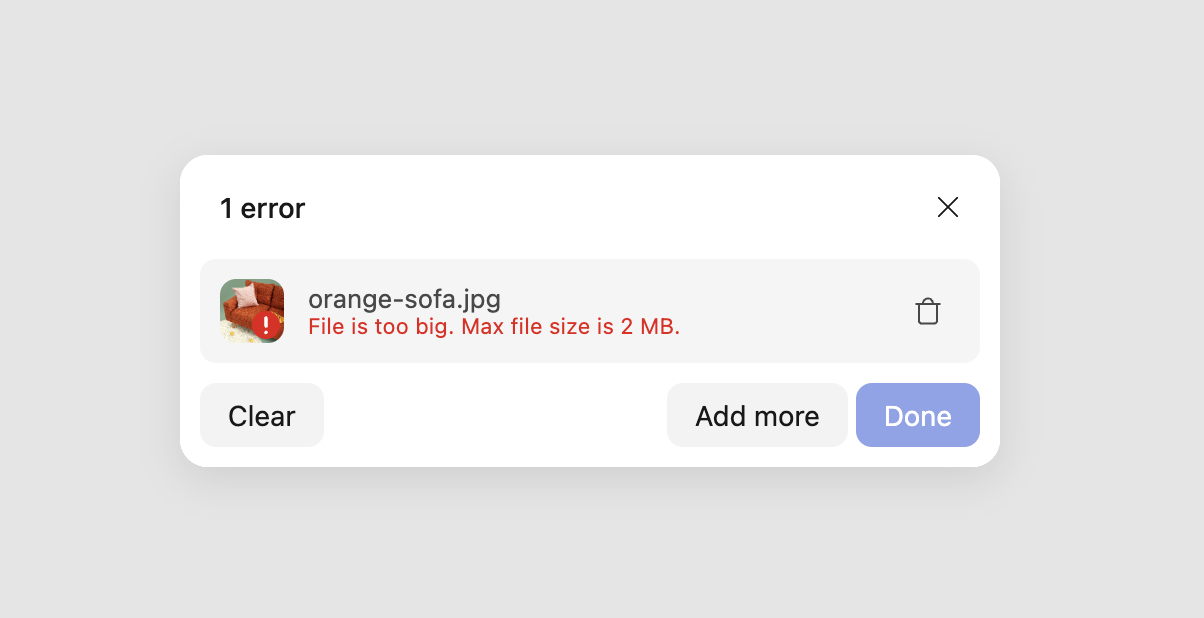
2. Custom validators
Custom validators allow you to define validation rules based on your specific needs, such as checking dimensions, file types, or other custom parameters.
Custom validators are pure functions that return an error message if the validation fails.
There are two types of custom validators:
- File validator: Validates an individual files.
- Collection validator: Validates a collection of files.
File validators
As the name suggests, file validators are used to validate individual files.
A file validator function takes two parameters: outputEntry
and api
.
The outputEntry
parameter contains information about the uploaded file, such as the file name, size, and type.
The api
parameter provides access to the File Uploader API, which can be used to perform additional actions or checks.
For the e-commerce images, let’s create a custom validator to check the image dimensions to ensure that all images uploaded are 1080×1080 pixels:
const imageDimensions = (outputEntry, api) => {
if (outputEntry.fileInfo &&
(outputEntry.fileInfo.imageInfo.width !== 1080
|| outputEntry.fileInfo.imageInfo.height !== 1080)) {
return {
message: 'Image width and height must be 1080px',
};
}
}
The imageDimensions
function checks the width and height of the uploaded image and returns an error message
if the dimensions do not match the required 1080×1080 pixels.
You can then attach the custom validator to the File Uploader configuration using the code below:
const config = document.querySelector('uc-config');
config.fileValidators = [imageDimensions];
With the custom validator in place, the File Uploader will now check the dimensions of any images uploaded and display an error message if the dimensions do not meet 1080×1080px dimension requirement.
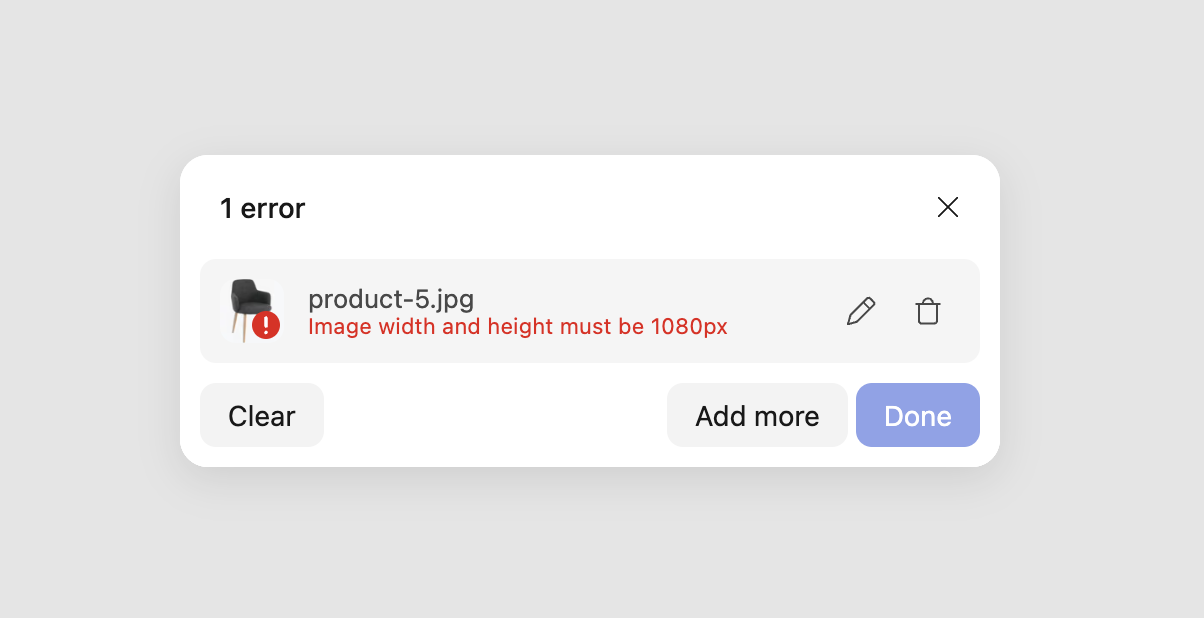
Collection validators
Collection validators are used to validate a set of files.
A collection validator function takes two parameters: collection
and api
.
The collection
parameter contains information about all the files, including their names, sizes, and types.
The api
parameter provides access to the File Uploader API, which can be utilized to perform additional actions or checks.
For example, let’s create a collection validator that checks the file names to ensure that an uploaded product image collection contains a front, back, and side images of the product. In this example we expect that file names include the word describing the view:
const hasAllViewAngles = (collection, api) => {
const hasFrontView = collection.allEntries
.some(file => file.isImage && file.name.includes('front'));
const hasSideView = collection.allEntries
.some(file => file.isImage && file.name.includes('side'));
const hasBackView = collection.allEntries
.some(file => file.isImage && file.name.includes('back'));
if (!hasFrontView || !hasSideView || !hasBackView) {
return {
message: 'Each product listing must include front, side, and back view images.',
};
}
};
The hasAllViewAngles
validator checks if the collection of files uploaded contains a front, back, and side images of the product for the e-commerce store.
If one image is missing, an error message is returned.
To apply the collection validator to the File Uploader, use the following code:
const config = document.querySelector('uc-config');
config.collectionValidators = [hasAllViewAngles];
With the collection validator in place, the File Uploader will now check for the required images in each product listing and display an error message if any images are missing.
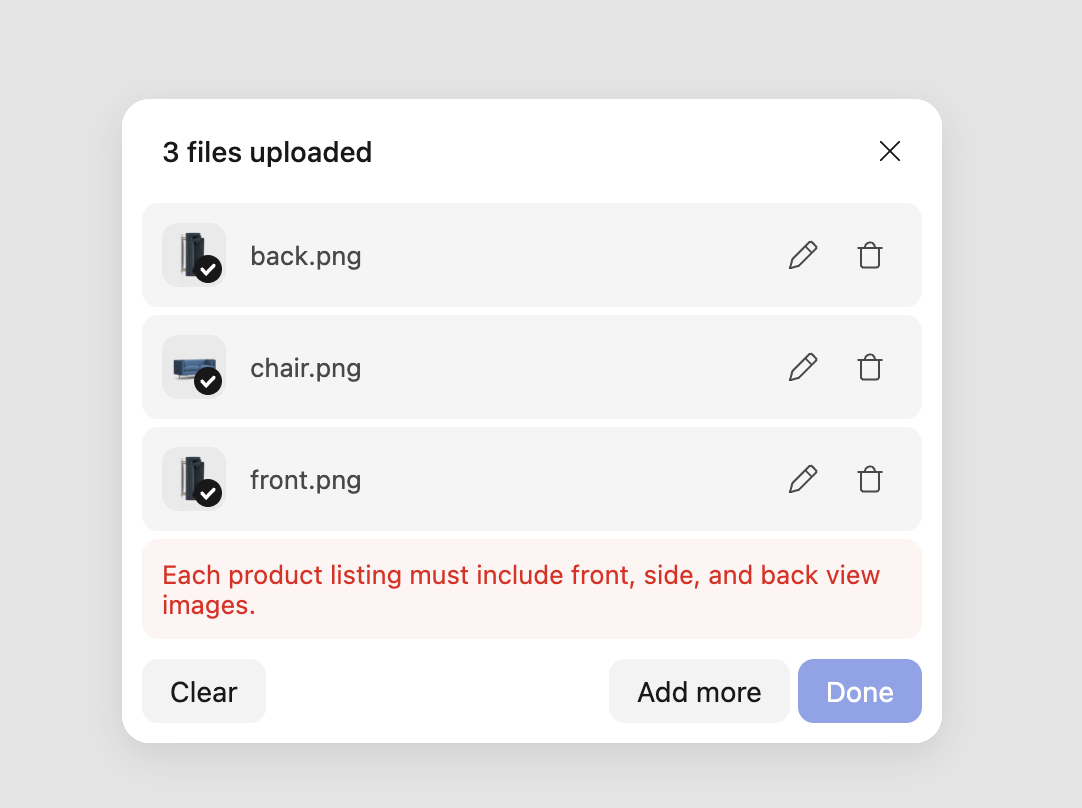
Displaying custom error messages
When you create a custom validator, it should return a custom message to display to users when a validation fails.
The validator function should usually return an object with a message
property set to the custom error message.
The message will be shown to the user when the validation condition is unmet.
For example, the imageDimensions
validator returns an error message if the image dimensions do not match the required 1080×1080 pixels:
{
message: 'Image width and height must be 1080px',
}
Localization of custom error messages
The File Uploader API allows custom error messages to be localized
if you already use localization in the File Uploader using the api
parameter in the validator function.
The api
parameter provides access to the l10n
function, which can translate error messages into different languages.
For example, to localize the error message for the imageDimensions
validator,
you can use the l10n
function to translate the message into different languages.
To do this, first, let’s import a localization file for the File Uploader just after importing the File Uploader components:
import es from 'https://cdn.jsdelivr.net/npm/@uploadcare/file-uploader@v1/locales/file-uploader/es.js';
After that, before defining the UC.defineComponents(UC)
, add the following code to define the localization for the File Uploader:
UC.defineLocale('es', {
...es,
custom: {
'image-dimensions-error': 'Las dimensiones de la imagen deben ser de 1080px',
},
});
Next, update the uc-config
component to use the es
locale:
<uc-config
locale-name="es"
ctx-name="my-uploader"
pubkey="YOUR_PUBLIC_KEY"
></uc-config>
With the localization defined, you can now use the l10n
function in the validator to translate the error message into the specified language.
For example, the imageDimensions
validator can be updated like this:
const imageDimensions = (outputEntry, api) => {
if (outputEntry.fileInfo &&
(outputEntry.fileInfo.imageInfo.width !== 1080
|| outputEntry.fileInfo.imageInfo.height !== 1080)) {
return {
message: api.l10n('image-dimensions-error'),
};
}
}
With this update, the error message for the imageDimensions
validator will be displayed in Spanish
when the image dimensions do not match the required 1080×1080 pixels.
Summary
File validators assist in validating files and setting restrictions for your project during the file upload procedure by utilizing both built-in and custom validators offered by our File Uploader tool. These validators enable you to enforce validation rules to uphold consistency and quality in your files. Whether you’re verifying image dimensions or checking file types and other parameters, validators offer a method to guarantee that all uploaded files adhere to the required criteria.